Script 25: Strategy Auto Assign on MTD Conv
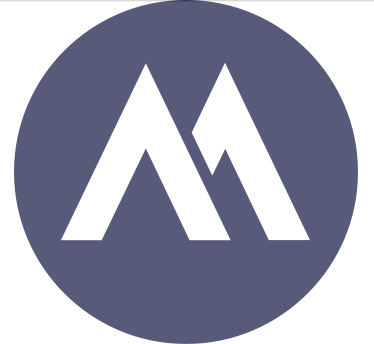
Purpose
The script reassigns marketing strategies to campaigns based on their Month-to-Date conversion levels.
To Elaborate
The Python script is designed to automatically reassign marketing strategies to campaigns by evaluating their Month-to-Date (MTD) conversion levels. It uses predefined conversion ranges to determine the appropriate strategy for each campaign. The script ensures that campaigns are aligned with the most suitable strategy based on their performance, optimizing resource allocation and potentially improving campaign effectiveness. The script applies a set of rules where each strategy is associated with a specific range of conversion values, and if a campaign’s conversion falls within a particular range, it is assigned the corresponding strategy. This reassignment process is crucial for maintaining an efficient marketing strategy that adapts to the dynamic performance of campaigns.
Walking Through the Code
- Define Strategy Conversion Ranges
- The script begins by defining a dictionary,
strategy_campaign_conversion_ranges
, which maps each strategy to a tuple representing the minimum and maximum conversion values. This dictionary serves as the basis for determining which strategy should be assigned to a campaign based on its conversion performance.
- The script begins by defining a dictionary,
- Initialize Data Structures
- Two new columns are added to the dataframes:
New Strategy
ininputDf
to track the newly assigned strategy, andStrategy
inoutputDf
initialized withnumpy.nan
to store the final strategy assignments.
- Two new columns are added to the dataframes:
- Apply Conversion Range Rules
- The script iterates over each strategy and its associated conversion range. For each campaign in
inputDf
, it checks if the campaign’s conversion count falls within the specified range. If it does, the campaign is assigned the corresponding strategy in theNew Strategy
column.
- The script iterates over each strategy and its associated conversion range. For each campaign in
- Identify and Output Changed Strategies
- The script identifies campaigns where the strategy has changed by comparing the original strategy with the newly assigned one. It prints out these campaigns, highlighting the changes in strategy.
- Update Output DataFrame
- The
outputDf
is updated to reflect the new strategies for campaigns that have undergone a strategy change. Only these campaigns are included in the final output, ensuring that the bulk file contains only relevant updates.
- The
Vitals
- Script ID : 25
- Client ID / Customer ID: 309909744 / 14196
- Action Type: Bulk Upload (Preview)
- Item Changed: Campaign
- Output Columns: Account, Campaign, Strategy
- Linked Datasource: M1 Report
- Reference Datasource: None
- Owner: Michael Huang (mhuang@marinsoftware.com)
- Created by Michael Huang on 2023-03-21 09:55
- Last Updated by Michael Huang on 2023-12-06 04:01
> See it in Action
Python Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
#
# Assign Campaign to Strategy according to Month-to-Date Conversions
#
#
# Author: Michael S. Huang
# Date: 2023-01-20
# define strategy conversion ranges
# if over-lapping, last rule wins
strategy_campaign_conversion_ranges = {
# key: strategy, value: (conv min, conv max)
'Unassigned': (0, 1000),
'Search - Non-Brand - Budget': (1000, 3000),
'Search - Top Performers': (3000, 5000),
'Full Funnel Bidding': (5000, 99999),
}
RPT_COL_CAMPAIGN = 'Campaign'
RPT_COL_ACCOUNT = 'Account'
RPT_COL_CAMPAIGN_ID = 'Campaign ID'
RPT_COL_STRATEGY = 'Strategy'
RPT_COL_CAMPAIGN_TYPE = 'Campaign Type'
RPT_COL_CAMPAIGN_STATUS = 'Campaign Status'
RPT_COL_DAILY_BUDGET = 'Daily Budget'
RPT_COL_PUB_COST = 'Pub. Cost $'
RPT_COL_COST_PER_CONV = 'Cost/Conv. $'
RPT_COL_ROAS = 'ROAS'
RPT_COL_AVG_CPC = 'Avg. CPC $'
RPT_COL_CONV_RATE = 'Conv. Rate %'
RPT_COL_CTR = 'CTR %'
RPT_COL_CONV = 'Conv.'
RPT_COL_REVENUE = 'Revenue $'
RPT_COL_SEARCH_LOSTTOPISBUDGET = 'Search Lost Top IS (Budget) %'
RPT_COL_SEARCH_LOSTTOPISRANK = 'Search Lost Top IS (Rank) %'
RPT_COL_LOST_IMPRSHAREBUDGET = 'Lost Impr. Share (Budget) %'
RPT_COL_LOST_IMPRSHARERANK = 'Lost Impr. Share (Rank) %'
RPT_COL_AVG_BID = 'Avg. Bid $'
RPT_COL_HIST_QS = 'Hist. QS'
RPT_COL_IMPR = 'Impr.'
RPT_COL_CLICKS = 'Clicks'
RPT_COL_PUBLISHER = 'Publisher'
BULK_COL_STRATEGY = 'Strategy'
outputDf[BULK_COL_STRATEGY] = numpy.nan
# add new column to track new strategy
inputDf['New Strategy'] = numpy.nan
# Apply conversion range rule for each strategy
for strat, (conv_min, conv_max) in strategy_campaign_conversion_ranges.items():
print("Applying Strategy Conv Range: ", strat, conv_min, conv_max)
inputDf.loc[ (inputDf[RPT_COL_CONV] >= conv_min) & (inputDf[RPT_COL_CONV] <= conv_max), 'New Strategy'] = strat
# find changed strategies
print("== Campaigns with Remapped Strategy ==")
changed = inputDf[ inputDf['New Strategy'].notnull() & (inputDf[RPT_COL_STRATEGY] != inputDf['New Strategy']) ]
cols = [RPT_COL_CAMPAIGN, RPT_COL_CONV, RPT_COL_STRATEGY, 'New Strategy']
print(changed[cols].to_string())
# copy new strategy to output
outputDf.loc[:,RPT_COL_STRATEGY] = inputDf.loc[:,'New Strategy']
# only include campaigns with changed strategy in bulk file
outputDf = outputDf[ inputDf['New Strategy'].notnull() & (inputDf[RPT_COL_STRATEGY] != inputDf['New Strategy']) ]
Post generated on 2024-11-27 06:58:46 GMT