Script 103: Tag Campaigns based on Name
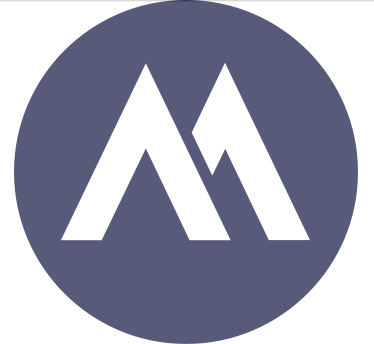
Purpose:
The script parses campaign names to extract and assign a tag based on a specific naming convention.
To Elaborate
The Python script is designed to parse campaign names and assign a tag to each campaign based on a predefined naming convention. The tag is extracted from the campaign name, specifically from the segment that appears after the first underscore (‘_’). This tag is then compared to an existing tag in the dataset, and if it differs, the new tag is assigned. The script ensures that only campaigns with non-empty and updated tags are included in the final output. This process helps in organizing and categorizing campaigns efficiently by adding a structured dimension tag, which can be useful for reporting and analysis purposes.
Walking Through the Code
- Configurable Parameters:
- The script begins by defining configurable parameters, such as the separator (
SEP
) used in campaign names and the location (TAG_LOCATION
) of the tag within the name. These parameters can be adjusted by the user to fit different naming conventions.
- The script begins by defining configurable parameters, such as the separator (
- Function Definition:
- A function
get_tag_from_campaign_name
is defined to extract the tag from the campaign name. It splits the campaign name using the separator and retrieves the tag based on the specified location. If the extraction fails, it returns an empty string.
- A function
- Data Processing:
- The script copies all input data to a new DataFrame for processing. It iterates over each row, extracting the campaign name and existing tag.
- Tag Assignment:
- For each campaign, the script extracts a new tag using the defined function. It compares this new tag with the existing tag, and if they differ, it updates the tag in the output DataFrame. If the tags are the same or the new tag is empty, it assigns a
NaN
value.
- For each campaign, the script extracts a new tag using the defined function. It compares this new tag with the existing tag, and if they differ, it updates the tag in the output DataFrame. If the tags are the same or the new tag is empty, it assigns a
- Output Filtering:
- The script filters the output DataFrame to include only rows with non-empty tags. It then prints the resulting DataFrame if it is not empty, ensuring that only relevant data is retained for further use.
Vitals
- Script ID : 103
- Client ID / Customer ID: 309909744 / 14196
- Action Type: Bulk Upload (Preview)
- Item Changed: Campaign
- Output Columns: Account, Campaign, Test
- Linked Datasource: M1 Report
- Reference Datasource: None
- Owner: Michael Huang (mhuang@marinsoftware.com)
- Created by Michael Huang on 2023-05-16 22:54
- Last Updated by dwaidhas@marinsoftware.com on 2024-02-01 15:58
> See it in Action
Python Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
#
# Add Dimensions Tag based on Campaign Name (minimal Pandas)
#
#
# Author: Michael S. Huang
# Date: 2023-05-17
#
########### Configurable Params - START ##########
SEP = '_'
TAG_LOCATION = 1 # comes after first separator
########### Configurable Params - END ###########
RPT_COL_CAMPAIGN = 'Campaign'
RPT_COL_ACCOUNT = 'Account'
RPT_COL_TEST = 'Test'
BULK_COL_ACCOUNT = 'Account'
BULK_COL_CAMPAIGN = 'Campaign'
BULK_COL_TEST = 'Test'
# define function to parse tag from campaign name
def get_tag_from_campaign_name(campaign_name):
vals = campaign_name.split(SEP)
# assume tag value is after 1st separator
tag = ''
try:
tag = str(vals[TAG_LOCATION])
except:
tag = ''
return tag
# copy all input rows to output
outputDf = inputDf.copy()
# loop through all rows
for index, row in inputDf.iterrows():
existing_tag = row[RPT_COL_TEST]
campaign_name = row[RPT_COL_CAMPAIGN]
tag = get_tag_from_campaign_name(campaign_name)
print("Campaign [%s] => Tag [%s]" % (campaign_name, tag))
# only tag if it's different than existing tag
if (len(tag) > 0) & (tag != existing_tag):
outputDf.at[index, BULK_COL_TEST] = tag
else:
outputDf.at[index, BULK_COL_TEST] = np.nan
# only include non-empty tags in bulk
outputDf = outputDf.loc[ outputDf[BULK_COL_TEST].notnull() ]
if not outputDf.empty:
print("outputDf", tableize(outputDf))
else:
print("Empty outputDf")
Post generated on 2025-03-11 01:25:51 GMT